October 22, 2023
Understand the Developer's Toolkit: Let's Break Down Tech Stacks
Navigate the world of programming technologies with this practical guide
The software development landscape is vast and ever-evolving. Keeping up with all the technologies involved in a modern product can feel overwhelming. This guide aims to break down the complexities, helping you understand what tech stacks are, why they matter, and how to navigate them effectively.
By the end, you'll have a clearer picture of the essential components, popular stack options, and key factors to consider when analyzing development toolkits.
Breaking down Tech Stacks: The Building Blocks
Think of a tech stack as a set of building blocks used to construct software applications. These blocks represent various technologies working together in layers, from foundational infrastructure to user-facing interfaces.
There are 2 main broad layers to build a product:
- Frontend: The user interface and interactive elements you see and interact with, often built with languages like JavaScript, HTML, and CSS.
- Backend: The server-side processing and data management, driven by programming languages like Python, Java, or Go.
Let's take an overview on how these layers could be presented together to make a deep dive in each sub-group:
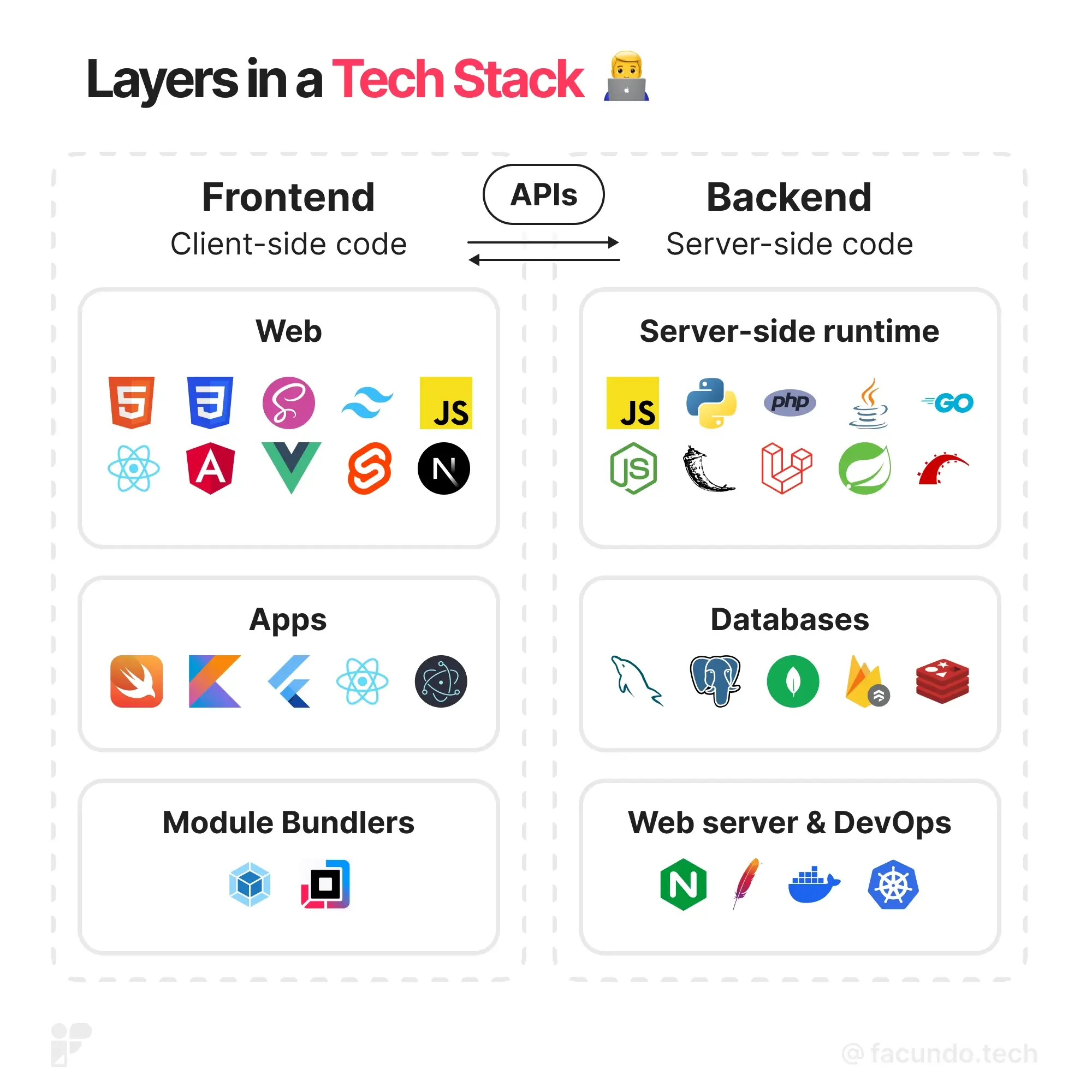
Its worth mentioning that tech stacks also are composed with additional components:
- Frameworks: Pre-built code libraries that accelerate development and provide structure.
- DevOps tools: Automation and collaboration tools that streamline software delivery and maintenance.
Frontend
Web technologies
The frontend of a website or web application is the part you see and interact with. It's your digital storefront, your virtual canvas, and it's built using a combination of essential technologies. Let's explore some of the key players:
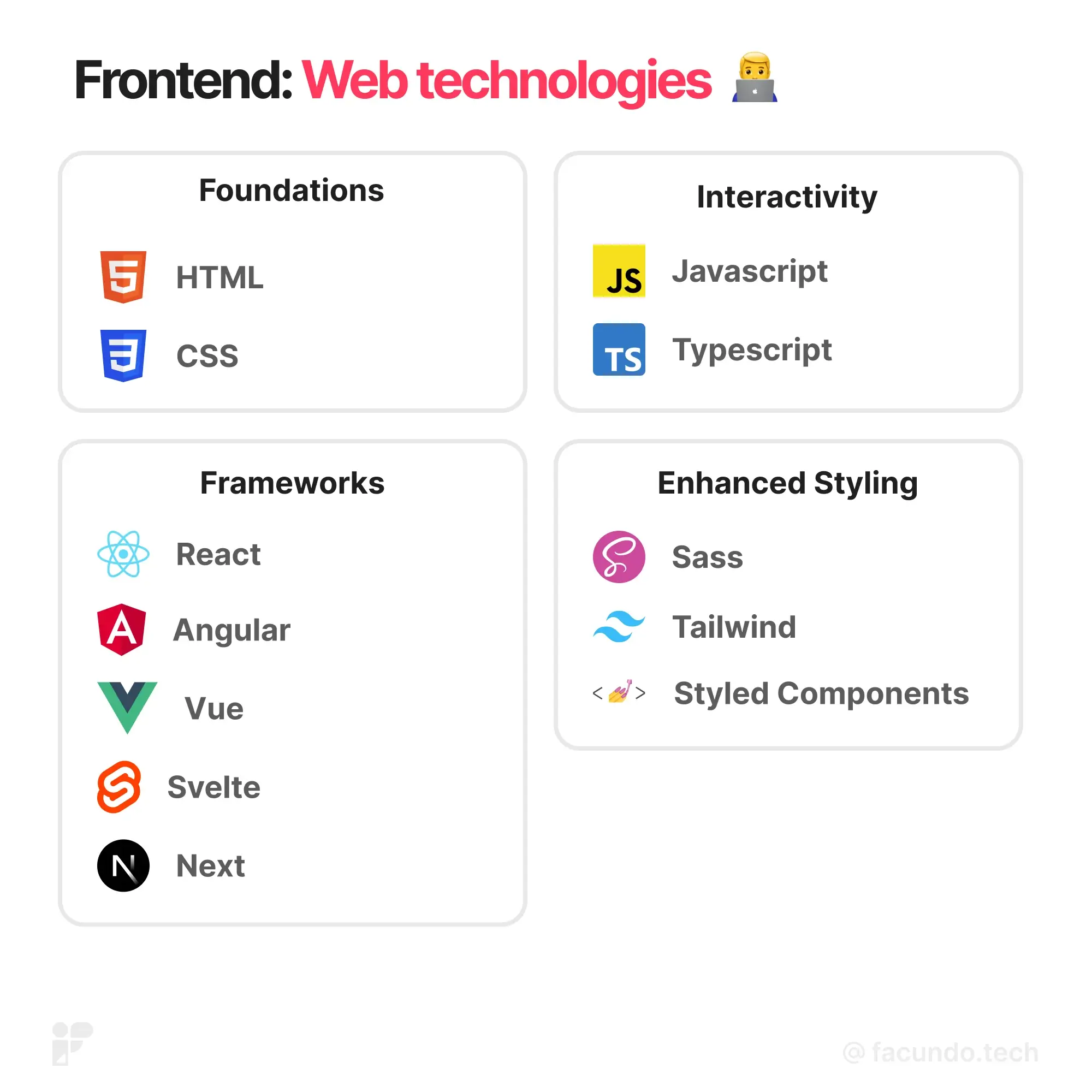
Foundations
- HTML (HyperText Markup Language): The backbone of web pages, defining the structure and content using tags like headings, paragraphs, and images. Think of it as the blueprint for your website's layout.
- CSS (Cascading Style Sheets): Makes websites visually appealing by controlling colors, fonts, layouts, and animations. It acts like the paintbrush and decorating tools that bring your HTML blueprint to life.
Interactivity and Dynamics
- JavaScript: The programming language responsible for interactivity and dynamic behavior. It's like the engine that powers animations, form validation, and user logins, taking your website beyond static content.
- Typescript:
Frontend Frameworks
- React, Angular, Vue, Svelte and Next: Build complex and dynamic user interfaces efficiently. Think of them as advanced construction tools that help you create interactive elements and complex functionalities with greater structure and maintainability.
Some important improvements when using these technologies include:
- Component-based architecture: Break down your interface into reusable building blocks, simplifying complex layouts and promoting code maintainability. Imagine dividing your website into modular Lego pieces for easier assembly and modification.
- Data binding: Automatically update UI elements based on changes in underlying data, streamlining dynamic displays and interactions. Think of it as having your UI components automatically adjust to reflect changes in real-time, like a speedometer reflecting your car's changing speed.
- Single-page application (SPA) capabilities: Create smooth and responsive user experiences without constantly reloading the entire page. Think of it as having a seamless, uninterrupted flow in your website even when navigating different sections.
These benefits on implementing these frameworks can be captured in:
- Increased Development Speed: Frameworks provide ready-made components and functionalities, reducing coding time and allowing developers to focus on core logic. Think of it as building your website with pre-fabricated elements instead of starting from scratch with bricks and mortar.
- Improved User Experience: Dynamic interfaces with smooth animations and responsive layouts enhance user engagement and satisfaction. Think of it as creating a user-friendly and enjoyable journey through your website, like navigating through a well-designed app.
- Scalability and Maintainability: Component-based architecture and modular coding practices make it easier to adapt and update your website as it grows, ensuring long-term sustainability. Think of it as having a well-organized house with defined sections that can be easily expanded or renovated without requiring a complete rebuild.
Enhanced Styling
- CSS Preprocessors (Sass, Less, Stylus): Extend CSS capabilities by adding features like variables, mixins, and nesting for cleaner, more manageable code. It's like having advanced brushes and color mixing tools for precise and efficient styling.
- CSS Frameworks (Tailwind, Bootstrap): Pre-built sets of styles and components that save you time and effort. Think of them as ready-made furniture sets for your website, offering quick customization options.
- CSS-in-JS (Styled Components, Emotion): Embed styles directly within your components, keeping your code organized and reducing reliance on separate CSS files.
- CSS Modules: Encapsulate styles within individual components, preventing accidental bleed-over and ensuring component-specific styling consistency. Think of each Lego piece having its own dedicated paint tray, preventing colors from mixing between elements.
Mobile and Desktop Apps
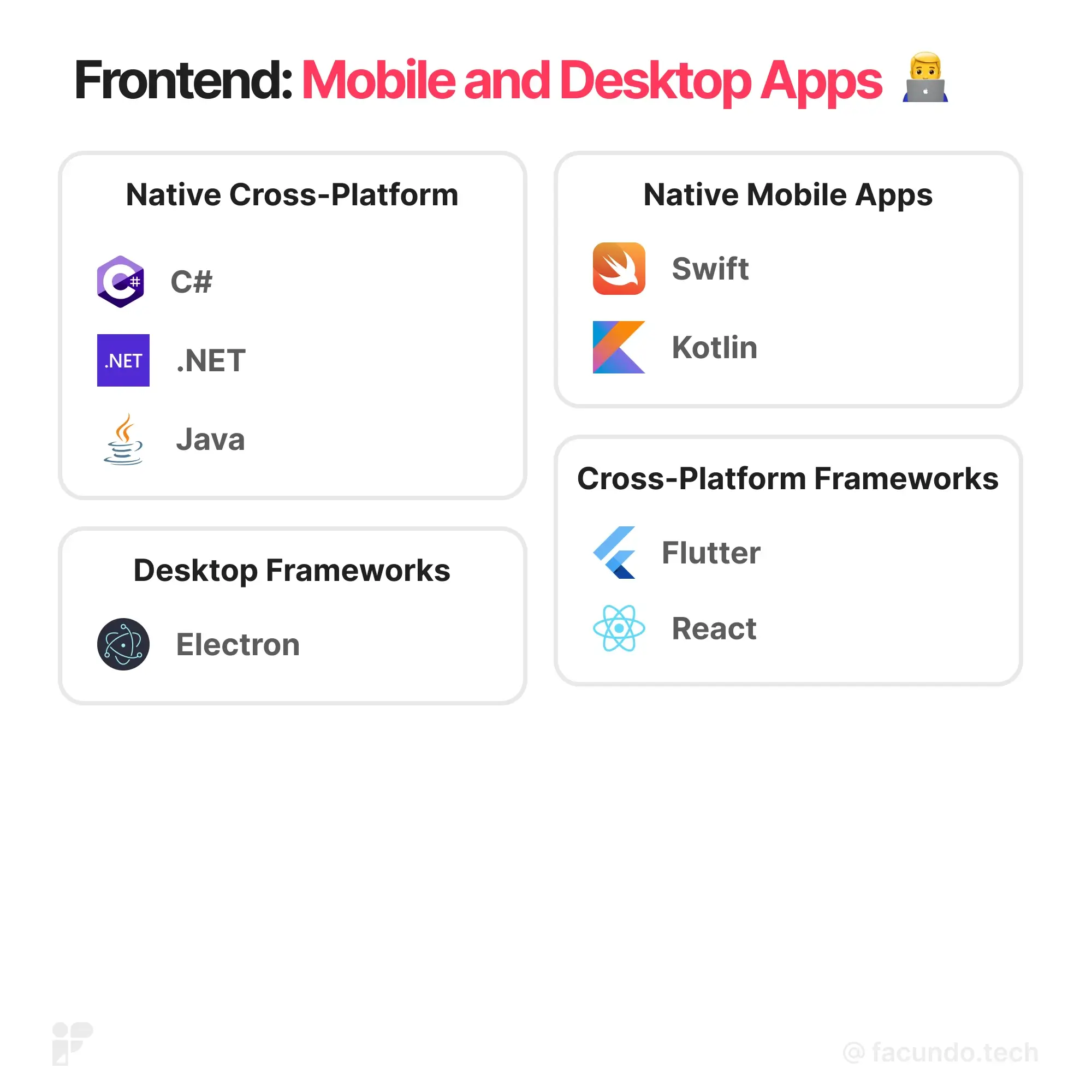
Native App Development
- C# and .NET: Craft a wide range of applications, from desktop software to web services, using Microsoft's powerful and versatile technologies. Think of them as a master toolkit for building both traditional homes and cutting-edge structures. Leverage Xamarin to create native Android and iOS apps with shared codebases, efficiently reaching multiple platforms with familiar tools. Imagine using a universal blueprint to construct houses that seamlessly adapt to different landscapes.
- Java: Develop cross-platform applications that run smoothly on various operating systems, known for its stability and extensive libraries. Think of it as a reliable construction material adaptable to different architectural styles.
Native Mobile App Development
- Swift (iOS): Apple's primary language for building smooth and intuitive iOS and iPadOS apps, known for its tight integration with native features and developer tools. Imagine it as crafting bespoke tools with precise Apple-approved materials.
- Kotlin (Android): Android's official language, offering conciseness, interoperability with Java, and powerful features for robust and modern Android app development. Think of it as building sturdy frameworks with advanced functionalities for the Android ecosystem.
- C# and .NET (Cross-Platform, with Xamarin for Native Android and iOS)
- Java (Cross-Platform)
Cross-Platform Frameworks
- Flutter: Google's framework allowing you to build beautiful and native-feeling apps for both iOS and Android using a single codebase. Imagine creating custom-made furniture that fits perfectly in both your living room and bedroom.
- React Native: Facebook's popular framework utilizing JavaScript and React principles to build mobile apps with reusable components and native UI elements. Think of it as assembling modular parts with familiar tools to create unique mobile experiences.
Note: Native performance vs. cross-platform efficiency: Native apps may offer superior performance, but cross-platform frameworks can save development time and resources.
Desktop App Development
- Electron: Frameworks like Electron combine popular web technologies like HTML, CSS, and JavaScript with powerful native APIs to build robust desktop applications for various platforms. Imagine using familiar building blocks like LEGOs to construct sturdy desktop software.
- C# and .NET
- Java
Module Bundlers
Module bundlers are development tools that organize and optimize JavaScript code for seamless delivery to web browsers. Popular options like Webpack and Turbopack excel in this area, streamlining your code into efficient bundles for production-ready deployment. Module bundlers achieve their task through several key functions:
- Combining Files: It merges numerous JavaScript files into a single, optimized bundle. This significantly reduces the number of HTTP requests required for loading, leading to faster page load times and a smoother user experience.
- Resolving Dependencies: It meticulously analyzes your code to identify and accurately link all necessary code modules, ensuring everything functions as intended without conflicts or missing components.
- Transforming Code (Optional): If your project utilizes cutting-edge JavaScript features, bundlers can transpile them into code compatible with older browsers, guaranteeing wider accessibility and compatibility across diverse user environments.
- Generating Dependency Graphs: As it traverses your code, a bundler constructs a visual map of dependencies, showcasing how different files and modules interrelate. This graph proves invaluable for maintaining code organization, identifying potential issues, and ensuring all files remain up-to-date and error-free throughout development and maintenance cycles.
Module bundlers often work alongside other helpful tools that further enhance your frontend development workflow:
- ESLint: Ensures consistent code formatting and identifies stylistic errors, keeping your code clean and easy to read. Imagine it as a code inspector ensuring everyone uses the same building codes for a uniform and efficient structure.
- Babel: Translates modern JavaScript syntax to work with older browsers, allowing you to leverage the latest features while maintaining compatibility. Think of it as a language translator ensuring all construction workers understand each other's instructions.
- PostCSS: Optimizes and transforms your CSS code, minimizing file size and improving performance. Picture it as a fashion consultant streamlining your CSS wardrobe for efficiency and clarity.
Backend
Server-side runtime
The frontend of a website or web application is the part you see and interact with. It's your digital storefront, your virtual canvas, and it's built using a combination of essential technologies. Let's explore some of the key players.
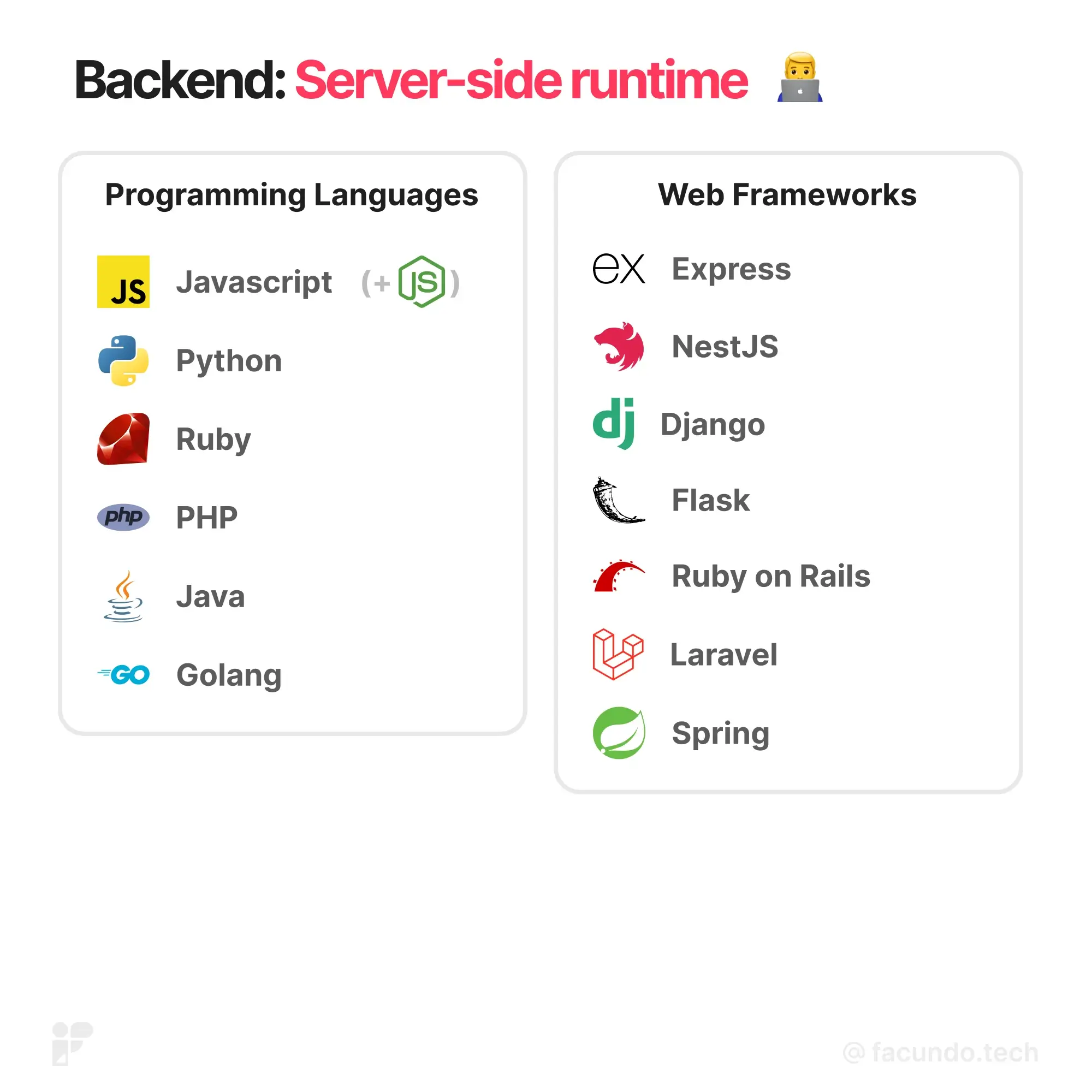
Programming Languages and their Runtime Environments
- JavaScript and Node.js: Node.js, JavaScript's runtime environment, enables server-side development and real-time applications, making it a popular choice for modern web projects.
- Python: Often executed within virtual environments like venv or virtualenv to manage dependencies and isolate project environments, Python excels in web development with frameworks like Django and Flask.
- Ruby on Rails: Ruby on Rails, a full-stack framework, includes its own runtime environment, streamlining web development with convention-over-configuration principles.
- PHP: Typically runs with web servers like Apache or Nginx, PHP powers content management systems like WordPress and frameworks like Laravel, providing mature toolsets and a vast community.
- Java: Usually executed within the Java Virtual Machine (JVM), Java's Spring framework offers a comprehensive toolkit for building robust and scalable web applications.
- Go: Go, a compiled language, doesn't require a traditional runtime environment. It directly compiles to machine code, known for its speed and efficiency in web development.
Web Frameworks
- Express: A minimalist and flexible framework for Node.js, Express offers core web application features and customization options, allowing for tailored solutions.
- NestJS: Built on top of Express, NestJS provides a structured and scalable framework with TypeScript support, promoting code organization and maintainability in large-scale projects.
- Django: A high-level Python framework known for its "batteries included" approach, Django offers a comprehensive set of features, streamlining development processes.
- Flask: A microframework for Python, Flask provides flexibility and control for building web applications with a minimalist approach, granting developers greater customization freedom.
- Ruby on Rails: A full-stack framework focused on convention over configuration, Rails emphasizes rapid development and clean code structure, enforcing best practices and efficient workflows.
- Laravel: A popular PHP framework known for its elegance and expressive syntax, Laravel offers a rich set of features and a vibrant community, providing a comprehensive development experience.
- Spring: A comprehensive Java framework for building enterprise-grade applications, Spring provides modularity and a vast ecosystem of tools and libraries, ensuring scalability and robustness.
Databases
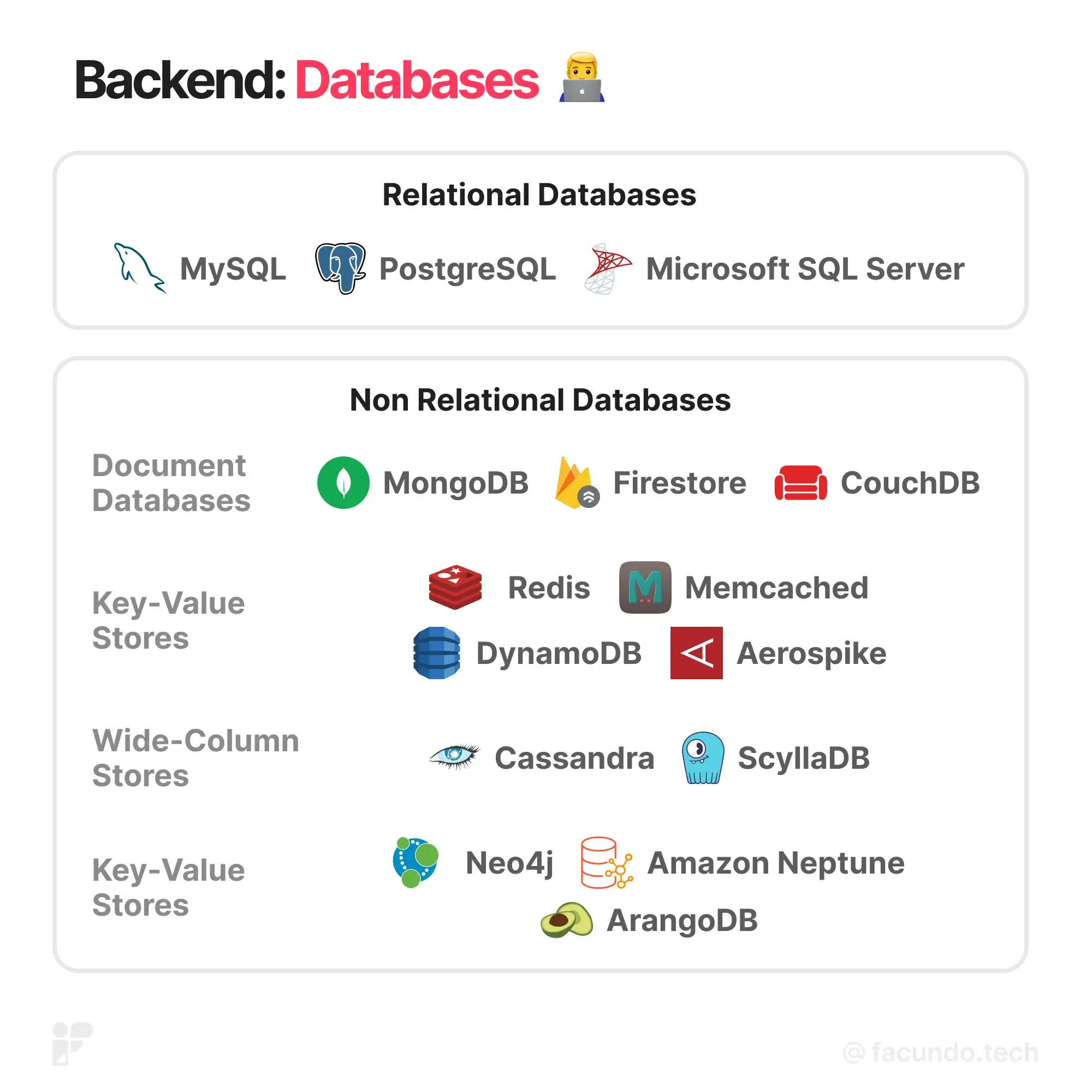
Relational Databases: Structured Data Storage
Relational databases organize data in tables with defined relationships, enabling precise search and retrieval through query languages (like MySQL, PostgreSQL, and Microsoft SQL Server). These databases excel at handling structured data like user accounts, product catalogs, and financial transactions, ensuring efficient indexing and reliable data connections.
Non-relational Databases: Flexibility for Unstructured Data
Sometimes, information doesn't neatly fit into tables and rows. Non-relational (often called NoSQL) databases offer flexibility for diverse data types. Popular options like MongoDB, Firestore, BigTable, Redis, and Cassandra come in several subcategories:
- Document Databases: Store data in flexible, document-like formats, ideal for user preferences, social media posts, or blog entries (examples: MongoDB, Firestore, CouchDB).
- Key-Value Stores: Organize data as simple key-value pairs, efficient for caching and real-time applications (examples: Redis, Memcached, DynamoDB, Aerospike).
- Wide-Column Stores: Handle large datasets with variable structures, suitable for time series data or sensor readings (examples: Cassandra, ScyllaDB).
- Graph Databases: Capture and explore complex relationships between entities, useful for social networks, recommendation systems, and fraud detection (example: Neo4j, Amazon Neptune, ArangoDB).
NoSQL databases excel at scalability, data manipulation ease, and handling diverse data types without rigid structures.
Web Servers and DevOps Tools
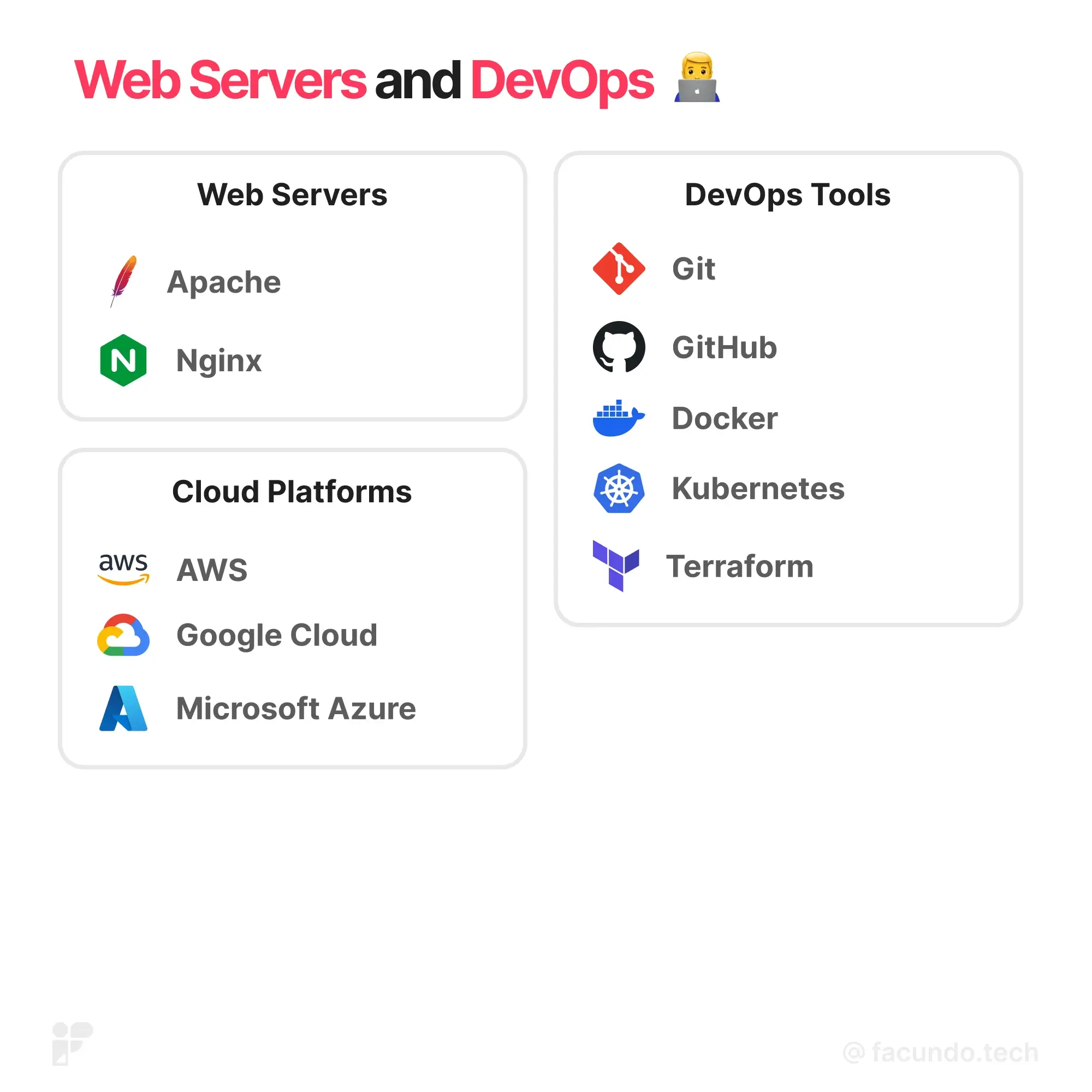
Web Servers
The web server receives requests from users, like accessing a webpage or downloading a file, and decides what information to send back. Popular options include:
- Apache: A mature and reliable choice, well-suited for high-traffic websites and complex configurations.
- Nginx: Known for its speed and efficiency, often favored for performance-critical applications.
DevOps Tools
DevOps tools bridge the gap between development and operations, streamlining website deployment, management, and collaboration. Key players include:
- Git: A version control system that tracks changes to your website code, enabling versioning, rollback, and collaborative development.
- GitHub/GitLab: Cloud-based platforms for hosting and managing Git repositories, facilitating access and collaboration among developers.
- Docker: Creates isolated containers for website code and its dependencies, ensuring consistent execution across different environments.
- Kubernetes: Orchestrates and manages multiple Docker containers, automating deployment, scaling, and resource allocation.
These tools work together to automate tasks, improve development speed, and ensure your website runs smoothly.
Cloud Platforms
Cloud platforms like Google Cloud, AWS, and Microsoft Azure provide on-demand infrastructure and services for hosting your website. They offer:
- Scalability: Quickly adjust resources like storage and computing power based on your traffic needs.
- Cost-efficiency: Pay only for the resources you use, avoiding high upfront investments.
- Global reach: Deploy your website in multiple regions for improved performance and availability.
Cloud platforms empower you to focus on website development and leave infrastructure management to the experts.
Infrastructure as Code
Infrastructure as code (IaC) is the ability to provision and support your computing infrastructure using code instead of manual processes and settings. Any application environment requires many infrastructure components like operating systems, database connections, and storage. Developers have to regularly set up, update, and maintain the infrastructure to develop, test, and deploy applications.
Manual infrastructure management is time-consuming and prone to error—especially when you manage applications at scale. Infrastructure as code lets you define your infrastructure's desired state without including all the steps to get to that state. It automates infrastructure management so developers can focus on building and improving applications instead of managing environments. Organizations use infrastructure as code to control costs, reduce risks, and respond with speed to new business opportunities.
Terraform takes infrastructure provisioning to the next level, defining infrastructure configuration in code. This code can then be used to automatically create and manage resources across different cloud platforms, leading to consistent and repeatable deployments.
Popular Tech Stacks for Diverse Needs
Each project has unique requirements, influencing the ideal tech stack. Here are some common options:
- LAMP (Linux, Apache, MySQL, PHP): A classic web development stack known for its simplicity and affordability.
- MEAN (MongoDB, Express.js, AngularJS, Node.js): A JavaScript full-stack solution favored for its speed and community support.
- MERN (MongoDB, Express.js, ReactJS, Node.js): Similar to MEAN, but leveraging ReactJS for its dynamic interface capabilities.
- Python Stack (Django, Flask, PostgreSQL): Popular for data-driven applications and API development, offering rapid prototyping and flexibility.
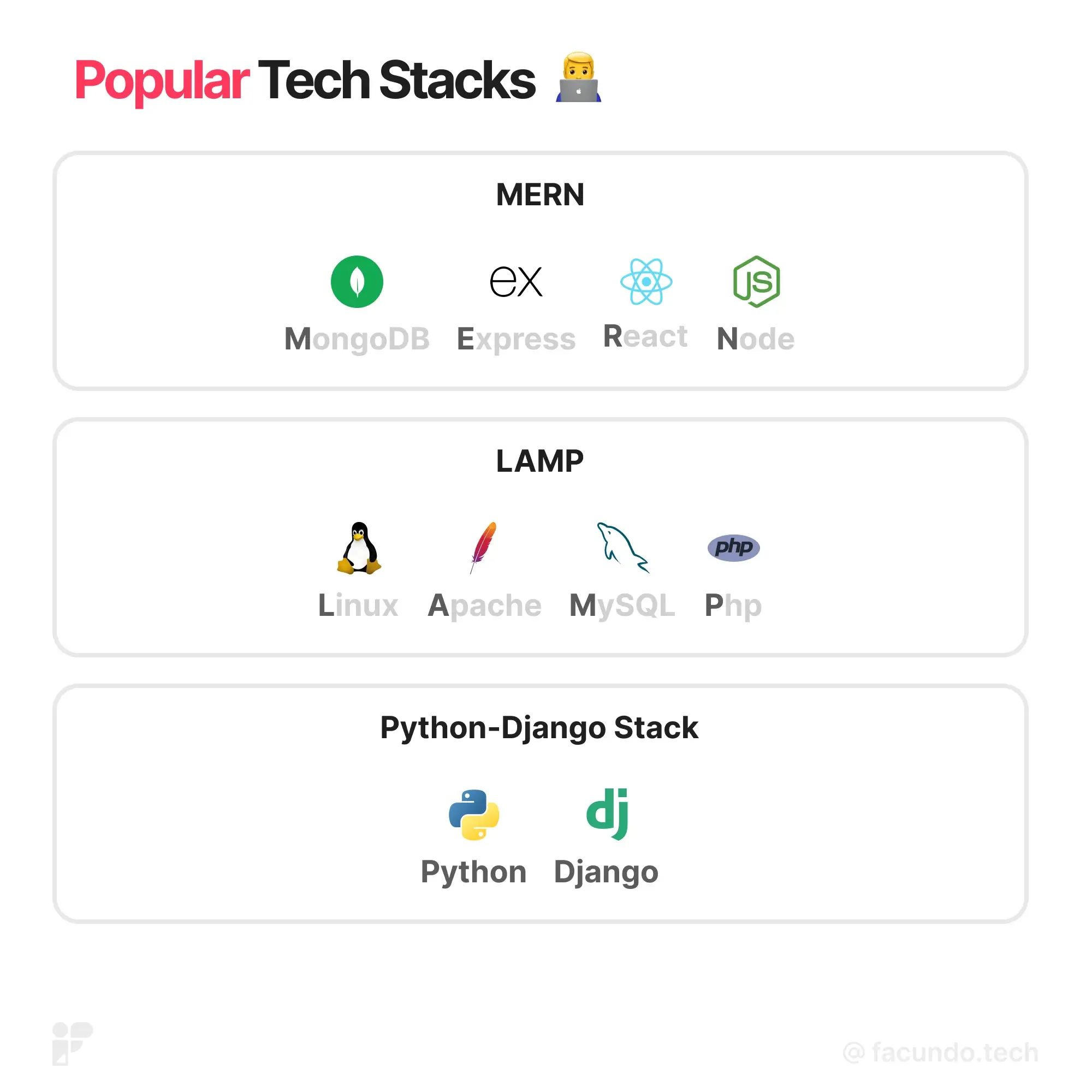
Choosing the Right Stack: Key Considerations
While popular stacks can be tempting shortcuts, selecting the right one hinges on several factors:
- Project requirements: Consider features, functionalities, and performance demands.
- Developer expertise: Choose technologies your team already knows or is comfortable learning. Scalability and maintainability: Ensure the stack can adapt to future growth and support ongoing updates.
- Community and resources: Access to documentation, tutorials, and active communities is crucial.
- Cost and complexity: Weigh the budget implications and learning curve associated with different options.
Remember, there's no "one-size-fits-all" solution. Research, experiment, and adapt your tech stack as your project and skills evolve.
Key Takeaways
- Tech stacks are sets of technologies used to build software applications.
- They comprise frontend, backend, database, and additional components.
- Popular stacks offer pre-built solutions, but choosing the right one depends on project needs, expertise, and other factors.
- Continuous research, experimentation, and adaptation are key to maintaining an effective tech stack.